· Travis Rodgers · Coding Tutorials · 3 min read
How to Display the Primary Category for a Product or Post In WordPress Loop
There will come a time where you will want to display the Primary Category for a Product or Post in a WordPress loop and only the Primary Category.
For example, a search results page and you want the entry meta of the results to display only the primary category.
First off, this is a Yoast construct, the idea of making a category a Primary Category, so we should keep that in mind when looking to display the Primary Category for a Product or Post.
So in this post, I want to show you how to do this and not only how to populate the Primary Category, but place it as a link in your entry meta.
Getting The Primary Category from a WooCommerce product
WooCommerce Products:
So in your WordPress loop you want to display WooCommerce Products with only the Primary Product Category displayed. And to add to that, you want that Category to be linked. Something like this:
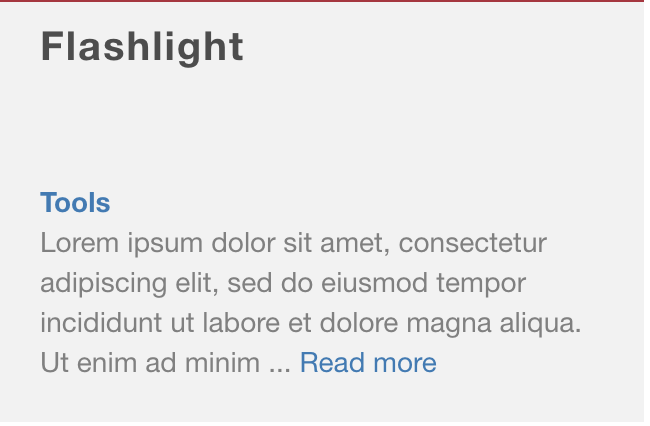
Well, here's the code to pull that Primary Category and populate it as a link. Place it inside your loop:
//Grabs the Primary Category of the Product
$primary_term_product_id = yoast_get_primary_term_id('product_cat');
$postProductTerm = get_term( $primary_term_product_id );
//If the post type is a product, display the Primary Category Link
if ( 'product' === get_post_type() ) { ?>
<div class="">
<?php
if ( $postProductTerm && ! is_wp_error( $postProductTerm ) ) {
echo '<a href="' . esc_url( get_term_link( $postProductTerm->term_id ) ) . $postProductTerm->slug . '">';
echo $postProductTerm->name;
echo '</a>';
}
?>
</div>
Getting The Primary Category from a Post
Posts:
And let's say you want to display only the Primary Category of a post, perhaps after the date. And to add to that, you want that Category to be linked. Something like this:
Well, here's the code to pull that Primary Category and populate it as a link. Place it inside your loop:
//Grabs the Primary Category of the Post
$primary_term_id = yoast_get_primary_term_id('category');
$postTerm = get_term( $primary_term_id );
//If the post type is a post, display the Primary Category Link
if( 'post' === get_post_type() ) { ?>
<div class=""><?php the_time('m/j/Y'); ?> | <?php
if ( $postTerm && ! is_wp_error( $postTerm ) ) {
echo '<a href="' . esc_url( get_term_link( $postTerm->term_id ) ) . $postTerm->slug . '">';
echo $postTerm->name;
echo '</a>';
}
?>
</div>
One Caveat…
Remember, your posts or products prior to Yoast implementing this feature in 2016 will not have a primary category so this will not work for those. In fact, it will leave a big, fat, blank space.
So you will need to return the category as normal.
Lets say there is no primary category and instead of returning them all you just want to return the first one. Well, you could put in an else to your if statement, targeting the first category in the array:
$postCategory = get_the_category();
else {
echo '<a href="' . esc_url( get_term_link( $postCategory[0]->term_id ) ) . $postCategory[0]->slug . '">';
echo $postCategory[0]->name;
echo '</a>';
}```
<p>Or let's say you want to do the same with the product category:</p>
```php<?php
$productTerms = get_the_terms( $post->ID, 'product_cat' );
else {
if ( $productTerms && ! is_wp_error( $productTerms ) ) {
foreach ($productTerms as $productTerm) {
if ($productTerm === reset($productTerms)) {
echo '<a href="' . esc_url( get_term_link( $productTerm->term_id ) ) . $productTerm->slug . '">';
echo $productTerm->name;
echo '</a>';
}
}
}
}
And that's it! Let me know if you have any questions!