· Travis Rodgers · Programming · 6 min read
How to Create Sticky Sidebar Ads in WordPress (No Plugin Needed)
I went through a phase last year where I ditched my sidebar. I liked the minimalist look and the effort to keep the reader focused on the article at hand and not be distracted by things in the sidebar.
I still think of it as a great strategy, but I eventually missed the sidebar and the great things it offered, so I brought it back.
But one thing that never sat right with me was all the empty space in long articles. Once your sidebar runs out, the rest of the article is left with a gaping space on the side of the screen.
And this is where I think sticky sidebar ads can serve a great purpose. Here's how it works:
Once the reader scrolls to the end of the sidebar, the last ad, or announcement, or whatever widget you put there, "sticks" to the side of the screen and scrolls on down the page with you.
Want an example? View this post on Desktop or Table, scroll down the page, and when you get to the last widget (currently an opt in for an ebook of mine) it will begin to scroll down the page with you. Then as you reach the bottom of the content it "releases" and pops back up the page to join the sidebar again.
I see a lot of people doing this with ads, but again, you can do this with anything.
How to create a sticky sidebar ad in WordPress
Here are the JavaScript ingredients:
1. Grab the ID of your widget and of your Content
In Chrome, right click on the element and click Inspect. This will open the Chrome Developer Tools. Find the section ID for your Element. I am using a Thrive Leads widget so mine would be widget_thrive_leads-4:
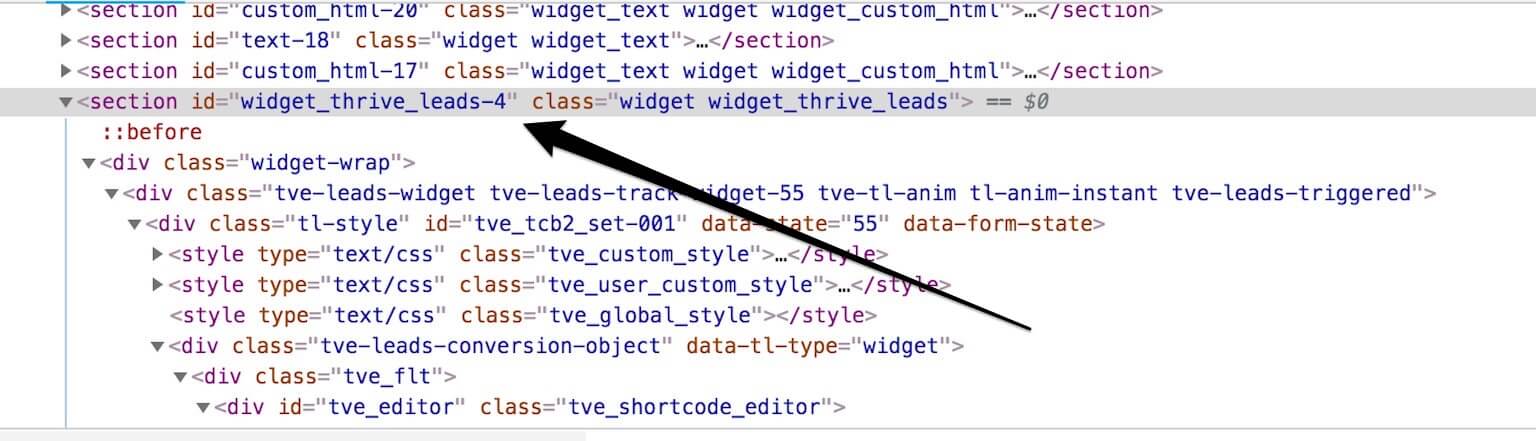
Do the same for your content area. There are usually two sections to your page: The content area, and the aside (sidebar). Grab the ID of the content area. In my case, it's genesis-content.

2. The code explained
There are couple of varables that you want to attain:
- Distance from the top of the page to the sidebar ad.
- The height of the entire content area.
- The height of the entire sidebar
- We'll use $(window).scroll to measure how far we've scrolled from the top.
Next, we only want this sticky sidebar ad to happen if the content is longer than the sidebar. And to be safe, we've added 1000px padding.
Next, if we've scrolled up to, or past, the distance to the sticky sidebar ad, we add the class 'sticky-ad' which creates the 'sticky' effect. Here's the CSS for that:
.sticky-ad {
position: fixed;
top: 10px;
max-width: 312px;
}
Be sure to set the max-width to whatever the max width of your sidebar widgets are.
Finally, we need to "release" the ad back to the sidebar when we reach the bottom of the content. So if our scroll height from the top of the page exceeds the content height, we remove the 'sticky-ad' class. I also put 1000 padding on this to be sure it releases a bit early.
3. The code
So with all that said, here's the code. You can place this in your JavaScript file or if you only want this script to load on blog posts you can put it in functions.php with the logic of:
if (is_single() && !wp_is_mobile()){?>
//JavaScript code goes here
<?php
}
And here's the full code (be sure to replace the widget and content variables with your own):
(function($) {
// After page has loaded so elements fall into place
$(window).on('load', function() {
// Distance from top of page to sidebar ad in px
var adFromTop = $('#widget_thrive_leads-4').offset().top
// Height of entire content area
var contentHeight = $('#genesis-content').height();
// Height of entire sidebar
var sidebarHeight = $('#genesis-sidebar-primary').height();
// Making sure my content is longer than my sidebar with 1000px padding
if (sidebarHeight < contentHeight + 1000) {
$(window).scroll(function() {
// If scroll distance from top exceeds by ad distance from top, add class
if ($(window).scrollTop() >= adFromTop) {
$('#widget_thrive_leads-4').addClass('sticky-ad');
} else {
$('#widget_thrive_leads-4').removeClass('sticky-ad');
}
// If scroll distance from top is greater than content height remove class. Added 1000px to pull ad out a bit before reaching the bottom.
if ($(window).scrollTop() > contentHeight - 1000) {
$('#widget_thrive_leads-4').removeClass('sticky-ad');
}
});
}
});
})( jQuery );
Why jQuery?
For this sort of thing, jQuery just works better. Yes I know the bloat of jQuery and 99% of the time will seek out a vanilla JS solution.
In this case, the heights with vanilla JS differed with different browsers causing the sticky sidebar ad to "stick" and "release" at bad times. jQuery was consistent in all, so I went with that.
If you prefer the vanilla JS solution for this, then here's the code to do it, but be sure to test and tweak for different browsers. For instance, it just didn't work well in Firefox.
document.addEventListener('DOMContentLoaded', function() {
var sidebarAd = document.getElementById('#widget_thrive_leads-4');
// When the user scrolls the page, execute the function below
window.onscroll = function() {adStick()};
// Get the navbar
var content = document.getElementById("genesis-content");
var scrollTop = window.pageYOffset || (document.documentElement ||
document.body.parentNode || document.body).scrollTop
// Get the offset position of the ad widget
var sticky = sidebarAd.offsetTop;
var contentHeight = content.offsetHeight;
// Add the sticky class to the navbar when you reach its scroll
position. Remove "sticky" when you leave the scroll position
function adStick() {
if (window.pageYOffset >= sticky - 40) {
sidebarAd.classList.add("sticky-ad")
} else {
sidebarAd.classList.remove("sticky-ad");
}
if (window.pageYOffset > contentHeight - 1000) {
sidebarAd.classList.remove("sticky-ad");
}
}
}, false);
Conclusion
And with a little bit of JavaScript we can add a sticky sidebar ad to our WordPress site without any plugin.
Let me know if you have any questions or want to contribute as to why the vanilla JS solution works differently in different browsers.