· Travis Rodgers · Programming · 3 min read
React Hook Form Controller v7 Examples (MaterialUI, AntD, and more)
I recently completed a React project that used react-hook-form and loved it. It’s a rock-solid way to get forms up and running quickly.
But it wasn’t long into the project before I realized I had to figure out how to integrate third-party UI Libraries with it (my Library of choice was Bulma).
Thankfully, React Hook Form has it all covered with their Controller component.
The React Hook Form Controller Component is a wrapper component that takes care of the registration process on third-party library components. It performs the backend magic so you can still partake in using the custom register.
There are already posts out there explaining the parts of the controller (as well as some great documentation), so I decided to help out by simply supplying working React examples.
React Hook Form Controller Examples
So this page exists to provide working examples for those looking to use the React Hook Form Controller component.
This is a living blog post. Please let me know what other libraries or components should be added or that you may be confused about and I’ll add it to the post.
Material UI
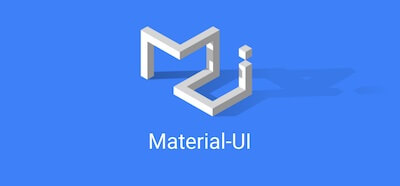
Switches
import React from 'react'
import { useForm, Controller } from 'react-hook-form'
import Switch from '@mui/material/Switch';
<Controller
control={control}
name='muiSwitch'
defaultValue={false}
render={({ field }) => (
<>
<Switch {...field} />
</>
)}
/>
Sliders
import React from 'react'
import { useForm, Controller } from 'react-hook-form'
import Slider from '@mui/material/Slider';
<Controller
control={control}
name='muiSwitch'
defaultValue={30}
render={({ field }) => (
<>
<Slider {...field} step={10} marks min={10} max={110}/>
</>
)}
/>
TextInput
import React from 'react'
import { useForm, Controller } from 'react-hook-form'
import TextField from '@mui/material/TextField';
<Controller
control={control}
name='muiTextField'
defaultValue="Hello World"
render={({ field }) => (
<TextField
{...field}
id="outlined-required"
label="Required"
/>
)}
rules={{ required: true }}
/>
{errors.muiTextField && <span>This date field is required</span>}
AntD
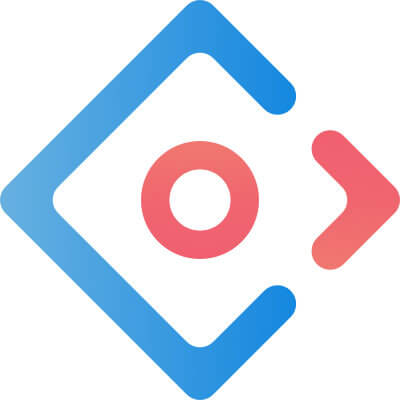
DatePicker
import React from 'react'
import { useForm, Controller } from 'react-hook-form'
import { DatePicker } from 'antd'
<Controller
control={control}
name='antdDatepicker'
render={({ field }) => (
<DatePicker
{...field}
onChange={e => {
field.onChange(e)
console.log(e['_d'])
}}
/>
)}
rules={{ required: true }}
/>
{errors.antdDatepicker && <span>This date field is required</span>}
Checkbox
import React from 'react'
import { useForm, Controller } from 'react-hook-form'
import { Checkbox } from 'antd'
<Controller
control={control}
name='antdCheckbox'
defaultValue={false}
render={({ field: { onChange, value } }) => (
<Checkbox onChange={onChange} checked={value}>
Checkbox
</Checkbox>
)}
/>
Select
import React from 'react'
import { useForm, Controller } from 'react-hook-form'
import { Select } from 'antd';
const { Option } = Select;
<Controller
control={control}
name='antdSelect'
render={({ field }) => (
<>
<Select defaultValue="lucy" {...field}>
<Option value="jack">Jack</Option>
<Option value="lucy">Lucy</Option>
<Option value="disabled" disabled>
Disabled
</Option>
<Option value="Yiminghe">yiminghe</Option>
</Select>
</>
)}
/>
Conclusion
As noted above, this is a living post. Let me know what should be added so we’ll have a resource example for React Hook Form Controller component examples.