· Travis Rodgers · Programming · 3 min read
Where Do I Store An API Key in WordPress?
Two ways to store an API key in WordPress:
1. In wp-config.php
Define the API key in the wp-config.php file. For example:
define( 'API_KEY', 'ieifkasdfasdf939390' );
This definition can be used anywhere without resorting to a global variable.
So when you need to use it just:
echo API_KEY;
Or simply use it as a variable where needed. For example:
$MailChimp = new \DrewM\MailChimp\MailChimp(API_KEY);
To further secure your wp-config.php file overall, check out my post on this: How to Easily Secure your wp-config.php File.
2. In the database
So for this example, I'll create an option in the WordPress admin for us to enter/update the API key to store in the database. In the end, it will look like this:
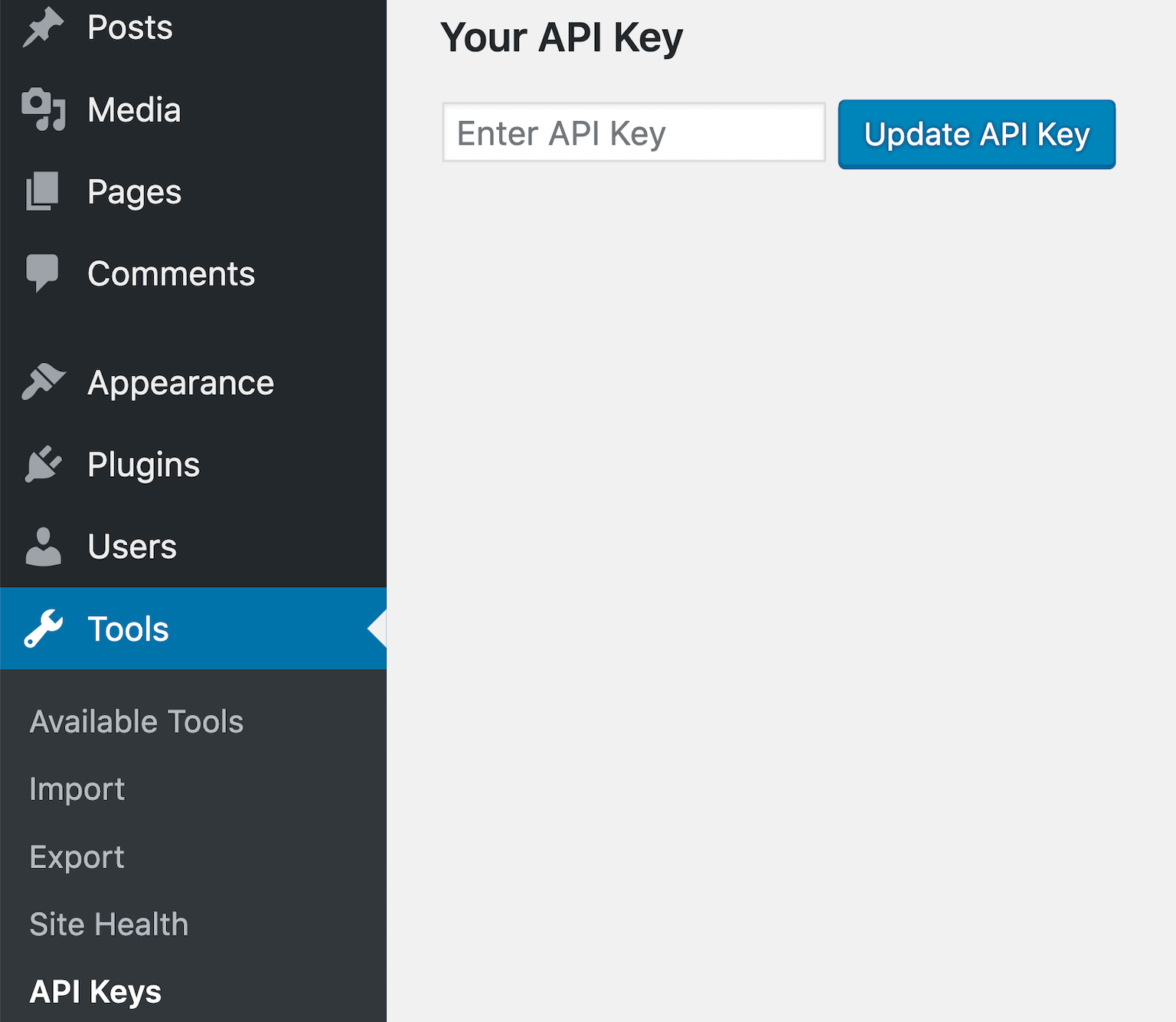
In the following code we will:
- Create an API Keys under the Tools section
- Create an admin page containing our form to enter they key
- The submit functionality to send the value to the options table
// Creates a subpage under the Tools section
<?php
add_action('admin_menu', 'wpdocs_register_my_api_keys_page');
function wpdocs_register_my_api_keys_page() {
add_submenu_page(
'tools.php',
'API Keys',
'API Keys',
'manage_options',
'api-keys',
'add_api_keys_callback' );
}
// The admin page containing the form
function add_api_keys_callback() { ?>
<div class="wrap"><div id="icon-tools" class="icon32"></div>
<h2>My API Keys Page</h2>
<form action="<?php echo esc_url( admin_url('admin-post.php') ); ?>" method="POST">
<h3>Your API Key</h3>
<input type="text" name="api_key" placeholder="Enter API Key">
<input type="hidden" name="action" value="process_form">
<input type="submit" name="submit" id="submit" class="update-button button button-primary" value="Update API Key" />
</form>
</div>
<?php
}
// Submit functionality
function submit_api_key() {
if (isset($_POST['api_key'])) {
$api_key = sanitize_text_field( $_POST['api_key'] );
$api_exists = get_option('api_key');
if (!empty($api_key) && !empty($api_exists)) {
update_option('api_key', $api_key);
} else {
add_option('api_key', $api_key);
}
}
wp_redirect($_SERVER['HTTP_REFERER']);
}
add_action( 'admin_post_nopriv_process_form', 'submit_api_key' );
add_action( 'admin_post_process_form', 'submit_api_key' );
Simply put, add_option adds our key to the database and update_options updates it when we already have a value there.
Now whenever we need to get access to the api key on our site we use:
get_option('api_key');
What about encryption?
Shouldn't we encrypt this key when we insert it into the database and decrypt it when we retrieve it?
We probably should, however, it's beyond the scope and purpose of this tutorial. If you'd like an example, let me know down in the comments below and I'll do a follow up post.
Conclusion
And there you have two ways to store an API key in WordPress.
Do you have a method by which you store api keys? I'd love to hear about it below.